Static
Static Variable and Static Method
- Static variable (also known as class variable)
- Static method (also known as class method)
public class S
{
private static int count = 0;
public S()
{
System.out.println("Constructor ...");
count++;
}
protected void finalize()
{
System.out.println("Finalize ...");
count--;
}
public static void main(String args[])
{
S s1 = new S();
S s2 = new S();
System.out.println("Count: "+S.count);
s1 = null;
s2 = null;
System.gc();
System.out.println("Count: "+S.count);
}
}
Static Block
- Static block is used for initializing the static variables
public class S
{
private static String name;
private static int year;
static
{
name = "Lin";
year = 37;
}
public static void main(String args[])
{
S s1 = new S();
System.out.printf("Name: %s\nAge: %d\n", S.name, S.year);
}
}
Static Nested Class
- Nested static class doesn’t need reference of Outer class
- A static class cannot access non-static members of the Outer class
- Able to access instance attributes and methods through an object reference
- As a member of the OuterClass, a nested class can be declared private, public, protected, or package private
- have access to other members of the enclosing class, even if they are declared private
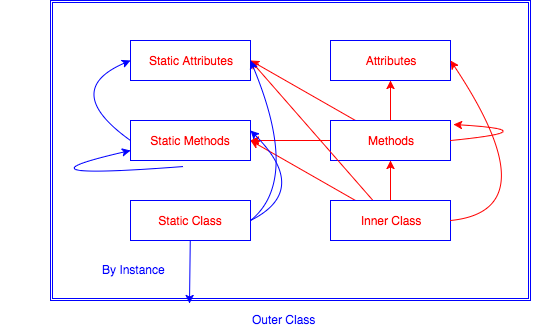
public class S
{
//non-static attribute
private int age;
//non-static methods
public int getAge() {return age;}
public void setAge(int a) {age = a;}
//static attribute
private static int count = 0;
//static method
public static int getCount() {return count;}
//static class
public static class C
{
private int year;
public C(int y) { year = y;}
public int getYear() { return year;}
//access static attribute and static method
public String toString() { return "C: "+year+" "+count+" "+getCount();}
//access outer class
public void display()
{
S s = new S();
s.setAge(20);
System.out.println("C: "+s.getAge());
}
}
}
public class STest
{
public static void main(String args[])
{
//create static class object
S.C c = new S.C(2017);
System.out.println(c);
c.display();
}
}
Static Import
- Enable you to refer to imported static members as if they were declared in the class that uses them
import static java.lang.Math.*;
public class S
{
public static void main(String args[])
{
System.out.printf("%10.2f\n", PI);
System.out.printf("%10.2f\n", sqrt(10.0));
}
}
Reference